Rapid API Prototyping a Virtual Lightbulb
Behind every sleek app and seamless digital experience lies a carefully crafted API. It’s hard to separate modern software from the API foundations it’s built upon. Businesses continue to expand their digital footprints, which requires more APIs — ideally constructed as quickly as possible. Rapid API prototyping could enable teams to move faster and collaborate better.
Driven by professional curiosity, I decided to experiment with a project that’s been on my mind for a while. For much of the last decade, a large marquee letter “D” illuminated the background of my Zoom calls. When asked, I would joke that it’s my “developer D light (delight).” What if I could show API principles through the operation of many virtual copies of that light, each person with their own API key to control their front-end view?
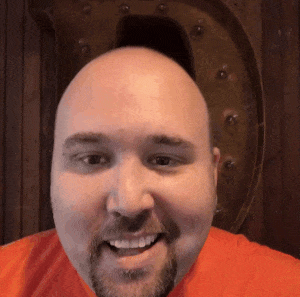
When the Ambassador team asked me to join the beta of their Blackbird API developer platform, it seemed like the perfect time to try out this project. Without a lot of time to devote to this sort of exploration, I knew rapid prototyping would enable me to see and share results faster.
Rapid prototyping is a manufacturing concept that has extended into software. The goal is to create functional representations as quickly as possible without spending time (and often money) making a final product.
In this post, you’ll see how I worked toward my end goal of a virtual series of lightbulbs as I rapidly prototyped the API to control them.
Start with an OpenAPI Description
In 2009, I joined ProgrammableWeb to follow and report on API trends. Back then, there were a few formats to describe APIs, most aimed at SOAP web services. Even then, it was clear the REST architectural pattern was the way forward compared to the SOAP standard — primarily because it allowed for much faster development and adoption. Eventually, the industry rallied behind the OpenAPI format to describe HTTP-based APIs. In 2024, there’s no doubt that any API project should start with OpenAPI.
There are many ways to create these description files (stored as JSON or YAML), but this is actually a great use case for Large Language Models (LLMs). These AI tools make a lot of sense to automate dev tool documentation. And OpenAPI is definitely a form of documentation.

The major LLM models understand OpenAPI formats, so you can use them to create the first version of your document. In my case, I started with Blackbird’s generation feature, likely powered by one of those LLM models behind the curtains. I described my use cases and some format requirements. It quickly responded with a draft — I browsed the description and accepted this first iteration.
Here’s how the endpoint that turns lights on and off looks in YAML format:
paths:
/lights/{lightId}:
post:
tags:
- Lights
summary: Turn a light on, off, or toggle its setting
operationId: controlLight
parameters:
- name: lightId
in: path
required: true
schema:
type: string
requestBody:
required: true
content:
application/json:
schema:
$ref: '#/components/schemas/LightControl'
Remember, we’re rapid prototyping here. API Design should include conversations with teammates about how endpoints and data are named. Naming continues to be the most challenging problem in software. But in the case of my lightbulb prototype, we can move on to feedback, another critical part of API Design.
Mock the API Endpoints
OpenAPI descriptions have become an essential part of the software workflow. Now that I have one for my light control API, I could use it to automate reference documentation, test live endpoints against a contract, and even SDK generation in multiple languages. However, this API is still just an idea with a description. It needs to become real — or the version of real that can be done quickly, with rapid prototyping.
When I generated the OpenAPI in the first place, Blackbird identified the four endpoints in my dashboard:

That view is much easier to interpret than reading an OpenAPI document, even when you know where to look. But neither tell the story that a live endpoint would give me. And, as a developer, I think in JSON.
Notice the second tab in Blackbird, which is where it provides my mock servers. Along with generating my OpenAPI document, Blackbird creates a live endpoint where I get dummy data about my new API.
For example, here is what’s returned when I call on the list of lightbulbs with the /lights endpoint:
[{
"lightId":"sunt mollit laboris nulla Duis",
"status":"on"
},
{
"lightId":"minim elit",
"status":"off"
}]
If I were working with someone else on the frontend, I could send them these working endpoints. They could build their own initial version — their own rapid prototype — of the light bulb dashboard.
This idea of mocking isn’t new, and Blackbird’s version has some deficiencies of your typical mock service. The dummy data is… pretty dumb. For example, the IDs are gibberish. It would be nice if they looked more realistic. This becomes especially noticeable when you get common fields like proper names. For what it’s worth, I’ve always liked how JSON Generator builds mock data. To do this, the tool requires much more context about the data than is available in an OpenAPI. Yet, I could imagine a future where the most common types of fields could be implied from the labels (ie, “name” or “email”).
When specific data is enumerated in an OpenAPI, as my lightbulb status values are, Blackbird’s mock generates good-looking data: “on” and “off” in this example. The best part is how mocking is built into Blackbird’s platform. I can hand off live endpoints for others to try out while I dive into the code that makes my API work.
Stub the API Control Code
At this point, my lightbulb API has been defined and deployed as a mock instance to a live URL without leaving the Blackbird dashboard. Now it’s time to “be a real developer.” That means I’m heading to my terminal’s command line interface (CLI).
It used to be that a dev tool’s CLI and web dashboard, if they both existed, felt like more separate experiences. It’s much more common now to expect to use each interface where it provides the least friction. Check out how easily Blackbird logs me in from the CLI and lets me get to the task:
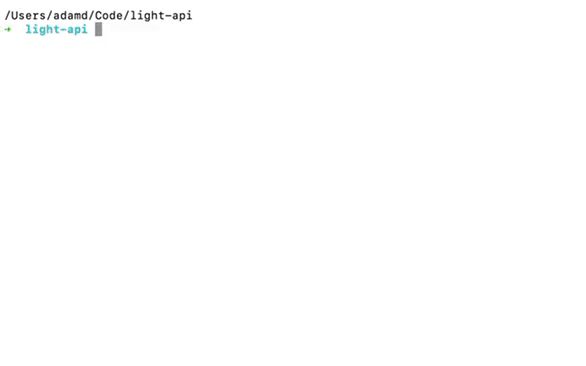
If this were my first login, I’d be bounced to my web browser for authentication (no tokens to copy-paste into the Terminal), then back with success.
The GIF also shows the files Blackbird generates for my API. They don’t include the logic for what data is returned, but the entire structure of my API — including the schemas defined in OpenAPI — is moved into boilerplate code. Right now, they only support GoLang, which has become a popular choice to build APIs. Ideally, there would be other language support. Granted, I could go find open source server generators, but I’d rather see it supported in this platform.
While this code generation might seem basic, imagine that most APIs usually have many more endpoints than the one I’ve created. Developers can handle a little copy-paste action for four similarly-named functions. That’s a much different story with a more extensive API.
In addition to code generation, Blackbird supports a lot of what the dashboard does — add an API (with an existing OpenAPI document) and create mock servers. I prefer the dashboard view since I can check the endpoints.
Once the code is written, you can run, debug, and deploy it. And that’s what’s left for me to complete my virtual lightbulb.
Build the Rest of the API
API-as-a-product trailblazers Twilio have a company value called “Draw the Owl.” It encourages employees to take action when there aren’t explicit directions. They’ll often share this humorous image to explain the idea:
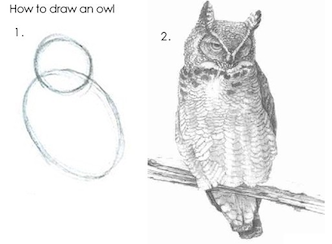
Step one is to draw a small circle on top of an oval. Step two is to “draw the rest of the owl.”
At this point in my project, I need to build the rest of the API. That may seem like a lot of work is ahead of me (and perhaps it is), but I got much farther than step one very quickly with Blackbird. I’ve created a rapid prototype for an API that didn’t exist 1,400 words ago. I have an OpenAPI description that I’ll use as I continue to build the API. My mock servers enable me, or anyone on my team, to build the frontend before the rest of the API is coded. Before I even start on my backend code, there are 679 lines I won’t have to write.
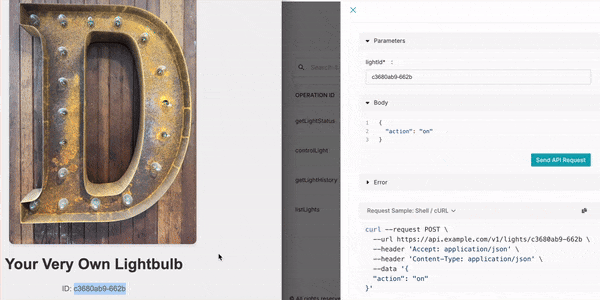
Yes, this is a prototype right now, but I will continue building. I’ll use a single-page app to show the state of any lightbulb. And I’ll build it on top of this new API.
If you want to create your own rapid API prototype, check out Blackbird, which is now out of beta — much appreciation to the team that let me have an early look.